Imagine having a web page where you can dynamically show or hide sections based on user interactions—clicks on buttons, headlines, or any triggers of your choice. This article delves into the fascinating world of creating interactive web content using simple HTML, JavaScript, and a touch of CSS. Whether you’re a web designer, developer, or just curious about enhancing user experiences, you’re in for an enlightening journey.
Using the Attributes ID and OnClick
One of the preferred methods for achieving this functionality is by using the attributes ID and OnClick. This approach offers simplicity, cross-platform compatibility, and ease of use. The following JavaScript code demonstrates a basic toggle function:
<SCRIPT>function ShowAndHide() { var x = document.getElementById(‘SectionName’); if (x.style.display == ‘none’) { x.style.display = ‘block’; } else { x.style.display = ‘none’; }}</SCRIPT> |
This code allows you to show or hide a designated section when a button is clicked:
<BUTTON ONCLICK=”ShowAndHide()”>Click me</BUTTON> |
You can replace the button with an image, link, or any other clickable element as needed. The section to be shown and hidden is typically represented by a DIV:
<DIV ID=”SectionName” STYLE=”display:none”>Text to be shown and hidden</DIV> |
Making It Visually Appealing
While the above solution is functional, it may lack visual finesse. To add a touch of elegance, you can use two buttons—each with its function. This approach separates the show and hide functions, making the code more organized:
<BUTTON ID=”Show” VALUE=”Click to show” ONCLICK=”ShowSection()”>Show section</BUTTON><BUTTON ID=”Hide” VALUE=”Click to hide” ONCLICK=”HideSection()”>Hide section</BUTTON><DIV ID=”SectionName” STYLE=” display:none”>Text to be shown and hidden</DIV> |
Using OnMouseOver for Hover Effects
OnMouseOver effects can add interactivity to your web content. However, this method may be less suitable for touch-based devices like tablets and smartphones. Nevertheless, let’s explore how to implement it:
<DIV ONMOUSEOVER=” ShowImage()” ONMOUSEOUT=”HideImage()”>Hold the cursor over this text!</DIV><IMG ID=”Logo” SRC=”your-image.png” ALT=”Image” STYLE=”display:none”> |
The JavaScript functions ShowImage() and HideImage() can be used to toggle the visibility of the image. This approach works well for creating hover effects, but may not be ideal for all scenarios.
Leveraging Radiobuttons for Exclusive Selections
Radiobuttons offer an exclusive selection mechanism, allowing only one section to be displayed at a time. This approach simplifies user interfaces when multiple choices are involved. Here’s a general solution:
<FORM STYLE=”padding-left:5px”> <INPUT TYPE=”radio” NAME=”RadioGroupName” ID=”GroupName1″ ONCLICK=”ShowRadioDiv(‘GroupName’, 3)”>Show section 1<BR> <INPUT TYPE=”radio” NAME=”RadioGroupName” ID=”GroupName2″ ONCLICK=”ShowRadioDiv(‘GroupName’, 3)”>Show section 2<BR> <INPUT TYPE=”radio” NAME=”RadioGroupName” ID=”GroupName3″ ONCLICK=”ShowRadioDiv(‘GroupName’, 3)”>Show section 3<BR></FORM> |
In this scenario, the JavaScript function ShowRadioDiv() ensures that only one section is visible at any given time. The sections to be displayed or hidden are represented by DIV elements with unique IDs.
Checkboxes for Multiple Choices
If you prefer a graphical representation of selections, checkboxes are a visually intuitive option. They are particularly useful when you have many sections to manage. Here’s how to create such functionality:
<FORM STYLE=”padding-left:5px”> <INPUT TYPE=”checkbox” ID=”BoxName1″ ONCLICK=”ShowCheckboxDiv(‘BoxName’, 3)”>Show section 1<BR> <INPUT TYPE=”checkbox” ID=”BoxName2″ ONCLICK=”ShowCheckboxDiv(‘BoxName’, 3)”>Show section 2<BR> <INPUT TYPE=”checkbox” ID=”BoxName3″ ONCLICK=”ShowCheckboxDiv(‘BoxName’, 3)”>Show section 3<BR></FORM> |
In this case, the JavaScript function ShowCheckboxDiv() manages the visibility of sections. The corresponding sections are defined using DIV elements with unique IDs.
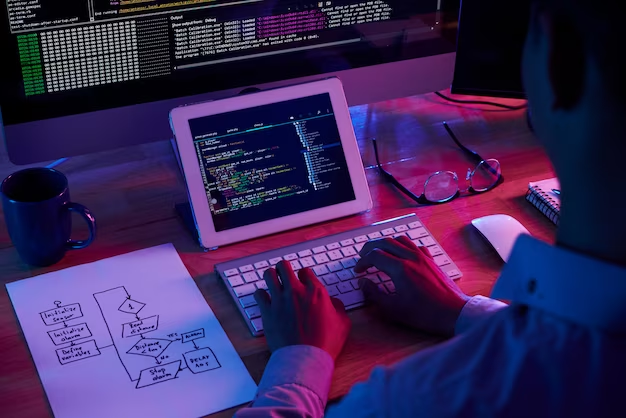
A Handy Comparison Table
Let’s summarize the different techniques for showing and hiding sections:
Technique | User Interaction | Suitable Devices | Use Cases |
---|---|---|---|
ID and OnClick | Button clicks | All devices | General use, simple interactivity |
OnMouseOver | Mouse hover | Desktops, some tablets | Hover effects, non-essential interactivity |
Radiobuttons | Radiobutton selection | All devices | Exclusive selections, limited user choices |
Checkboxes | Checkbox selection | All devices | Multiple selections, user-driven interactions |
Tips for Optimizing Hidden Click to Show Functionality
Now that you’ve learned how to implement hidden click to show functionality, let’s explore some advanced tips to optimize your interactive web content further:
- Smooth Transitions with CSS
Enhance the user experience by adding smooth transitions when showing or hiding sections. Utilize CSS transition properties to create elegant fade-ins, slides, or other visual effects. These transitions make your content more visually appealing and user-friendly.
#SectionName { transition: opacity 0.3s ease-in-out;} |
- Mobile-First Consideration
Given the prevalence of mobile devices prioritize mobile-first design. Ensure that your hidden click to show elements are responsive and work seamlessly on smaller screens. Test thoroughly across various devices to guarantee a consistent experience.
- Accessibility Matters
Make your interactive content accessible to all users, including those with disabilities. Utilize ARIA roles and attributes to provide meaningful information to screen readers. Ensure that keyboard navigation works correctly, and focus styles are visible for clickable elements.
- Code Optimization
Keep your code clean and organized. Remove redundant scripts, minimize external dependencies, and use efficient coding practices. This optimization will improve page loading times and overall performance.
- User Feedback
Provide clear visual feedback when users interact with your hidden click to show elements. Change button styles, display success messages, or use animations to confirm user actions. Feedback enhances the user’s understanding of the system’s response.
- Testing and Debugging
Thoroughly test your interactive elements across different browsers and devices. Use browser developer tools for debugging and ensure that there are no issues with functionality or display.
- Progressive Enhancement
Consider using progressive enhancement techniques. Start with basic functionality that works on all devices and browsers, and then add advanced features for modern browsers and powerful devices. This approach ensures a broader reach.
- User Analytics
Implement user analytics to gain insights into how users interact with your hidden click to show elements. Analyzing user behavior can help you make data-driven decisions for further improvements.
- Security Considerations
If your interactive content involves data retrieval or user authentication, prioritize security. Protect user data and ensure that your scripts are not vulnerable to common web security threats like XSS or CSRF attacks.
- Documentation
Maintain clear and comprehensive documentation for your interactive elements. This documentation will be valuable for future reference, troubleshooting, and collaborating with other developers.
Video Guide
To finally answer all your questions, we have prepared a special video for you. Enjoy watching it!
Conclusion
Creating interactive web content with 1 hidden click to show functionality empowers you to offer dynamic user experiences. Whether you choose the simplicity of buttons, the elegance of hover effects, the exclusivity of radiobuttons, or the versatility of checkboxes, the key lies in understanding how to manipulate HTML, JavaScript, and CSS to meet your design and functionality goals. So, dive in and enhance your web projects with the power of 1 hidden click to show!
FAQ
The hidden click to show functionality allows you to create interactive web content that can display or hide sections on a web page based on user interactions, such as clicks or hover events.
Hidden click to show functionality is supported on a wide range of devices, including desktop computers, laptops, tablets, and smartphones.
Radiobuttons are ideal for scenarios where you want to allow users to make exclusive selections. They ensure that only one section is visible at a time, simplifying user interfaces.
Yes, checkboxes are suitable for scenarios where you want to allow users to make multiple selections. They provide a visually intuitive way to manage multiple sections.
Yes, OnMouseOver may have limitations on touch-based devices like tablets and smartphones, as they lack traditional mouse input. In such cases, it’s important to consider alternative interaction methods.