Popups are a crucial part of web development, enhancing user interaction and providing valuable feedback. In this article, we’ll delve into the world of JavaScript popups using the alert(), confirm(), and prompt() functions. We’ll explore how to use them effectively to create dynamic and engaging user experiences on your website.
JavaScript Popups: Enhancing User Interaction
Popups play a crucial role in web development, enabling meaningful interactions with users. They serve various purposes, such as conveying error messages, soliciting user decisions, or collecting input. In essence, there are four primary types of popups you’ll encounter in web development:
- Alert Box: The alert box is commonly used to display informative messages to users. It contains a message and an “OK” button, allowing you to provide essential information or warnings;
- Confirm Box: The confirm box serves as a dialog for obtaining user feedback in the form of a binary response (“OK” or “Cancel”). This makes it ideal for confirming actions or preferences;
- Prompt Box: Prompt boxes are designed for gathering user input. They include a text input field alongside “OK” and “Cancel” buttons. You can use them to collect data from users;
- Modal Box: While not a specific JavaScript command, modal boxes are a versatile and widely used form of popups. They offer additional features and customization options not available with alert, confirm, and prompt boxes. Modal boxes are commonly employed in modern websites to enhance user interactions and create dynamic content overlays.
It’s worth noting that modal boxes have gained popularity due to their versatility and the ability to seamlessly integrate interactive content within web pages. As a result, many contemporary websites opt for modal boxes to provide a more engaging user experience. To explore modal boxes in more detail, refer to the dedicated page on our website.
Alert Boxes: Engaging User Notifications
Alert boxes serve as essential popup notifications that demand user attention and interaction. When presented with an alert box, users have a single option: clicking “OK” to acknowledge the message. In JavaScript, you can initiate an alert box using either the syntax window.alert() or simply alert().
Here’s an example of how to implement a button that triggers an alert message in code:
<BUTTON TYPE="button" ONCLICK="alert('Hello there!')">Click me!</BUTTON>
On screen, it looks like this:
Click me! |
Formatting options are quite limited with the alert function. You can only create a line break using ‘\n’. Let’s examine an example:
<BUTTON TYPE="button" ONCLICK="alert(' Hello there! \n Welcome!')">Click me!</BUTTON>
appearing as follows on the screen:
Click me! |
While it’s not possible to display images in an alert box, some individuals have devised creative workarounds. If you can present text, you can also create ASCII art. With a touch of creativity and patience, you can achieve something along these lines:
<BUTTON TYPE="button" ONCLICK="alert(' HELLO! \n\n (\\__/) \n (=\'.\'=) \n(\'\')__(\'\')')">Click a cat!</BUTTON>
resulting in the following appearance on the screen:
Click a cat! |
Please note that if you intend to display a backslash and a single quote as plain text rather than code, you must include a backslash before each, like so: ‘\\’ and ‘\’.
Confirm Boxes: User Verification
Confirm boxes, as the name suggests, are popup dialog boxes designed for user verification or confirmation. In contrast to the alert box, which offers a single “OK” button, the confirm box provides users with two options: “OK” and “Cancel.” This dual-choice dialog is commonly employed when you need users to confirm an action, such as opening a specific document or proceeding with a critical operation. Typically, clicking “OK” triggers one action, while selecting “Cancel” leads to an alternative course of action, or in some cases, no action at all.
The dynamic nature of the confirm box, which responds differently based on the user’s choice, necessitates the use of an if/else construct in your JavaScript code to handle the outcomes. While it’s possible to implement this directly using the onclick attribute, such an approach can become unwieldy as your codebase grows. Instead, the recommended practice is to create a separate JavaScript function that encapsulates the logic and then call this function when needed.
In the example provided here, the usage of a confirm box within an internal script is demonstrated for illustrative purposes. However, in practical scenarios, it’s often advisable to employ an external script for enhanced maintainability and organization. Confirm boxes are a valuable tool for engaging users in decision-making processes and ensuring that actions are taken deliberately.
The initial step is to have the button call the ‘confirmFunction()’ script. This can be achieved as follows:
<BUTTON TYPE="button" ONCLICK="confirmFunction()">Click me!</BUTTON>
Next, a script is created to change the grey area surrounding the button to green when the user clicks ‘OK’ (i.e., when ‘confirm’ equals true). Otherwise, it ensures the area retains its default grey color. The text prompting the user to click ‘OK’ or ‘Cancel’ is positioned after the ‘confirm’ function, with the text ‘Do you want a green background?’ utilized in this instance. To enable the code to function correctly, it is essential that the <div> containing the button has been assigned the ID ‘ColorChanger.’ This allows us to utilize ‘getElementById()’ in the example, resulting in the following code construction:
<SCRIPT>
function promptFunction() {
var Text = prompt("Write text here:", "");
if (Text != null) {
if (Text != "") {
document.getElementById("TextString").innerHTML = "You wrote " + Text + " in the field!";
} else {
document.getElementById("TextString").innerHTML = "You didn't write anything in the field!";
}}
else {
document.getElementById("TextString").innerHTML = "";
}
}
</SCRIPT>
<DIV ID="TextString"> </DIV>
When viewed on the screen, it will appear as follows:
Click me! |
Similar to the alert box, you’re limited to using text with the confirm box. Therefore, if you desire any graphical elements, you’ll need to resort to ASCII art.
Prompt Boxes: User Input and Interaction
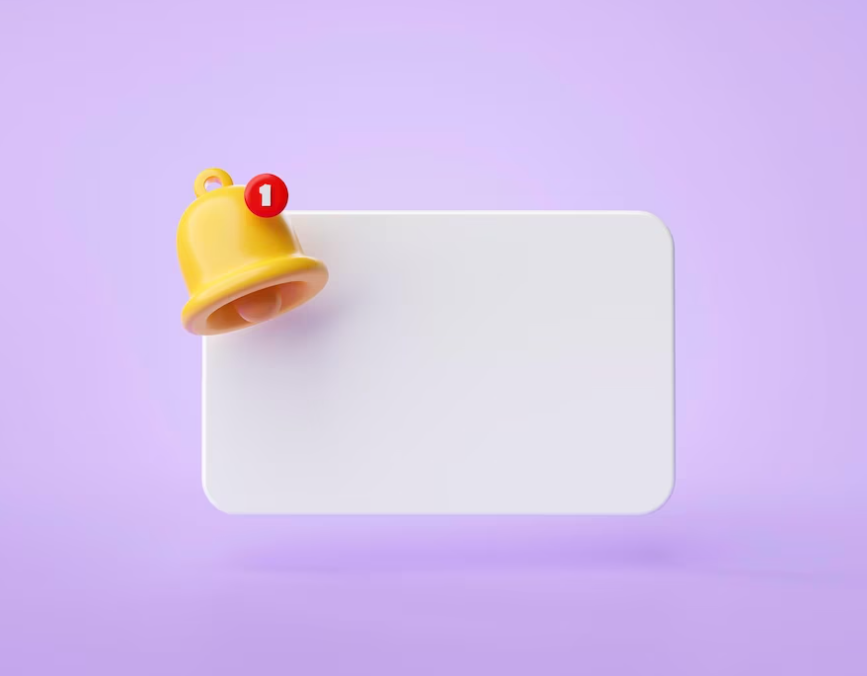
A prompt box serves as a user-friendly input dialog, making it convenient for users to provide various types of input, such as numeric values for calculations or access codes for authentication. It functions as an alternative to traditional input fields, enhancing the interactivity of your web applications. You can initiate a prompt box using the syntax window.prompt() or simply prompt(), depending on your coding preferences.
Just like the confirm() function, prompt() requires a JavaScript script to handle the input provided by the user effectively.
For the example presented here, a text input field is demonstrated, but it’s important to note that the input could just as easily represent an access code or a search term for a database query. Thus, the presence of a visible input field, as shown in the example, is not a strict requirement.
To get started, the first step is to create a button that triggers the execution of the promptFunction() script. The corresponding code appears as follows:
<BUTTON TYPE="button" ONCLICK="promptFunction()">Click me!</BUTTON>
Additionally, a text input field is necessary. In this instance, a <div> element identified by the ID ‘TextString’ is utilized for this purpose. Below is the code for the input field:
<DIV ID="TextString"> </DIV>
In addition to the text displayed within the prompt box created with prompt(), this function also allows the inclusion of a default value. These two text strings are enclosed in quotes and separated by a comma, as shown here: prompt(“The text in the box”, “The default value in the input field”).
Upon the appearance of the popup box, you are presented with two buttons, ‘OK’ and ‘Cancel,’ akin to the confirm() function. It’s worth noting that clicking ‘Cancel’ does not halt the script execution; instead, it returns the value null as your input. This distinction is crucial for structuring your script effectively.
To manage the content entered in the input field, one handles it as a variable—in this example, it is referred to as ‘Text.’ This results in the following code structure:
<BUTTON TYPE="button" ONCLICK="promptFunction()">Click me!</BUTTON>
<SCRIPT>
function promptFunction() {
var Tekst = prompt("Write text here:", "");
}
</SCRIPT>
<DIV ID="TextString"> </DIV>
In this particular example, differentiation between a click on ‘OK’ or ‘Cancel’ is not direct. Instead, an if/else construct is employed to check whether the variable ‘Text’ differs from null. If the user clicks ‘OK,’ the routine further distinguishes whether the input field contains text or is empty.
When there is text in the input field, it is appended to a text string. Conversely, if the field is empty, a message is triggered, notifying the user that they didn’t enter anything into the field. In the case of a ‘Cancel’ click, an empty string, “”, is assigned, ensuring that the script responds to the click without generating an error. This results in the following code structure:
<BUTTON TYPE="button" ONCLICK="confirmFunction()">Click me!</BUTTON>
<SCRIPT>
function confirmFunction() {
if (confirm("Do you want a green background?") == true) {
document.getElementById("ColorChanger").style.backgroundColor = "green";
} else {
document.getElementById("ColorChanger").style.backgroundColor = "#C0C0C0";
}
}
</SCRIPT>
When viewed on the screen, it will appear as depicted below:
Click me! |
How To Make A Popup Using HTML, CSS And JavaScript | Create a Modal Box In HTML Website
Conclusion
JavaScript popups like alert(), confirm(), and prompt() are vital for enhancing user interaction and feedback in web development. Alert boxes deliver messages, confirm boxes provide user verification, and prompt boxes enable input. Modal boxes are versatile options for creating dynamic content overlays, especially favored in modern websites. These popups collectively improve the user experience in web development.