In the ever-evolving landscape of web development, there exists a dynamic interplay between the creative use of code and the desire to craft captivating web experiences. Among the many tools in a web developer’s arsenal, the humble document.write() function stands as a venerable yet often underappreciated gem. This unsung hero of JavaScript and HTML allows developers to inject dynamic content and HTML code directly into a web page, facilitating the creation of interactive, responsive, and engaging web applications.
In this article, we embark on a journey through the realm of document.write(), delving into its capabilities, use cases, and potential pitfalls. Whether you’re a seasoned developer looking to expand your toolkit or a novice eager to grasp the fundamentals, join us as we explore the magic of document.write() and learn how it can transform static web pages into dynamic canvases of user engagement.
Exploring JavaScript for Web Text and HTML
JavaScript is a versatile language that empowers web developers with multiple ways to integrate and manipulate text within websites. This article dives deep into the fundamental concept of using JavaScript to display it, leveraging the document.write() method. This not only simplifies the process but also offers stability and flexibility. We’ll explore how to write basic text, add HTML elements dynamically, and provide useful tips for handling complex constructs.
Simple Text Display
Let’s begin with the basics – displaying it using JavaScript. The document.write() function makes it effortless. Here’s an example:
<SCRIPT TYPE="text/javascript">
document.write("Text with JavaScript");
</SCRIPT>
On the screen, it will appear as:
Text with JavaScript
Tip: To maintain code clarity, consider using indentation and line breaks when working with document.write(). This practice makes your code more manageable and minimizes errors.
Incorporating HTML Elements
JavaScript’s power truly shines when combining text with HTML elements. Take a look at how you can include HTML formatting within your text:
<SCRIPT TYPE="text/javascript">
document.write("Text with <I>JavaScript</I>");
</SCRIPT>
On the screen, it will appear as:
Text with JavaScript
Italics: JavaScript
Handling Quotation Marks
One challenge with integrating text and JavaScript is dealing with quotation marks. Since quotes delineate text, inserting them within the text can cause issues. However, a simple solution exists – using a backslash (“) before the quote:
<SCRIPT TYPE="text/javascript">
document.write("Text with \"JavaScript\"");
</SCRIPT>
On the screen, it will appear as:
Text with "JavaScript"
Pro Tip: To avoid confusion and improve code readability, consider using single quotes (‘ ‘) when specifying attributes within HTML elements.
Complex Constructions with document.write()
For more complex structures, such as tables, it’s advisable to break the code into several lines. While not mandatory, this practice enhances code manageability and minimizes errors. Let’s look at creating a basic table with document.write():
<SCRIPT TYPE="text/javascript">
document.write("<TABLE>");
document.write("<TR> <TD> </TD> <TD> </TD> </TR>");
document.write("</TABLE>");
</SCRIPT>
This code generates a table with one row and two cells. Structuring your code in this way makes it easier to understand and modify as your projects grow in complexity.
Recommendations for Using document.write():
- Always include the TYPE attribute when defining a script tag: <SCRIPT TYPE=”text/javascript”>;
- Use indentation and line breaks to maintain code clarity and readability;
- When adding HTML elements dynamically, ensure proper nesting and formatting for an organized display;
- Remember to escape quotation marks (“) within your text to prevent syntax errors;
- For intricate constructs like tables, consider breaking the code into multiple lines to simplify debugging and maintenance;
- Incorporating these best practices into your JavaScript text-writing endeavors will enhance your web development capabilities and streamline your coding experience.
Combining Text with Calculated Values and Variables in Scripting
When developing web content, there may be instances where it’s essential to merge static text with dynamic, calculated values or variables. This process ensures a more personalized and up-to-date user experience, displaying real-time data such as dates or user-specific information.
Embedding Dynamic Data within Static Text
To merge static text and dynamic values, the ‘+’ operator is used. For instance, when embedding today’s date after the static text “Today’s date is,” the code can be constructed as demonstrated below:
<SCRIPT TYPE="text/javascript">
let currentDate = new Date();
let day = currentDate.getDate();
let month = currentDate.getMonth() + 1; // Months are zero-based, hence the +1
let year = currentDate.getFullYear();
let formattedDate = month + "/" + day + "/" + year;
document.write("Today's date is " + formattedDate);
</SCRIPT>
When executed, this script dynamically calculates and combines the current date with the preceding text, rendering the following display on the screen:
Today’s date is 25/9/2023
Integrating Multiple Texts and Variables
If the composition necessitates more text or variable integrations, simply continue appending them using the ‘+’ operator. This method allows for the seamless incorporation of various data types and text, catering to more intricate and detailed content needs.
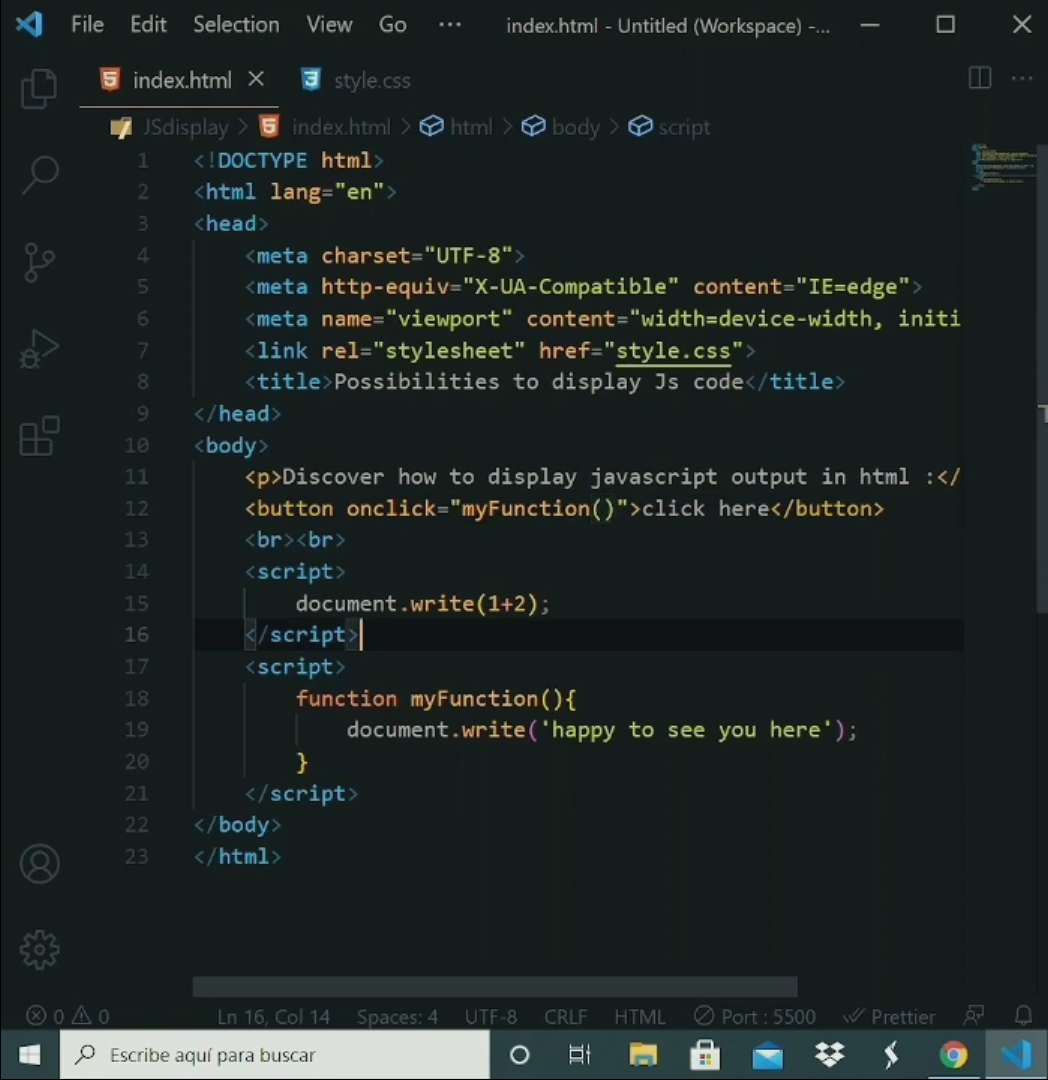
Enhancing Content with Dynamic Values
Employing such a technique not only enhances the content’s relevance but also its overall utility and user engagement. By integrating real-time or user-specific data, the displayed information remains current and more pertinent to the user, thereby creating a richer and more informative user experience.
Customization and Personalization
This method offers an ample opportunity for customization and personalization, allowing developers to tailor the content according to user behavior, preferences, or other contextual data, fostering a more intuitive and user-friendly environment. It is particularly significant in applications where timely and accurate information display is crucial, such as financial applications displaying stock prices, or travel applications showing real-time flight status.
Diverse Applications and Expanded Utility
While the given example illustrates the integration of date values, this methodology can be extended to a myriad of applications, each serving different purposes. Whether it’s appending user names to welcome messages or integrating real-time metrics to analytics reports, the amalgamation of static text with dynamic values contributes significantly to creating a comprehensive, detailed, and valuable user interface.
Conclusion
Ultimately, the choice of whether to use document.write() should depend on the specific requirements of a project and the trade-offs involved. Developers should carefully consider the implications and explore alternative approaches to ensure their web pages are performant, accessible, and maintainable in the long run. By staying informed about the latest web development techniques and best practices, developers can make informed decisions about when and how to use document.write() and other methods to create compelling and user-friendly web experiences.